Creating Variables and Using the JavaScript Alert(
Post# of 259
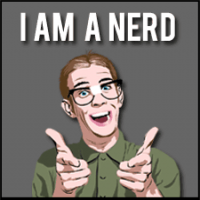
Creating Variables and Using the JavaScript Alert() Function
Let's create a simple HTML document that we can use to test our variables:
(Right click this link and choose to save the document to download a copy of this skeleton HTML file.)
You're going to want to have a better-defined HTML document when you're actually writing code, but for our purposes this will work just fine. Save the code above as a file called myscript.html (or anything you want that ends in .html and doesn't contain spaces or special characters) and open it up in your web browser. You'll see absolutely nothing other than "My Script" in the title bar. We still have more work to do. First, let's declare a variable inside of the script tag:
Here we've just declared a number. Let's look at other variable types we can declare
myString = "Hello world!";
myBoolean = true;
That gives us a number, a string, and a boolean. Now let's take that myString variable and actually do something with it:
myString = "Hello world!";
myBoolean = true;
alert(myString);
You'll notice I've added the line alert(myString);
. This calls a built-in JavaScript function (we'll learn more about these later) called alert()
which creates a pop-up dialogue box for users to interact with.
What is "Hello world!" all about?
Writing a simple program that says "Hello world!" is generally the first thing every programmer does when they're learning how to code. It's not necessary, but it's sort of a tradition and an initiation into the club.
You never really want to use these in practice because—as any internet user likely knows—alert boxes are very annoying, but they make for a good way to test your code, to make sure it works, while you're writing. The parenthesis following alert allow you to provide alert with data it might need. Not all functions will require that you give it information, but alert needs to know what to alert the user. In this case, we gave it myString, so the user will receive a popup notification that says "Hello world!" Try this with your other variables to get popups with a number and a boolean value.
Why give alert() a variable and and not just give it the contents of the variable? Well, if you said alert("Hello world!") you'd get the same result in this example, but variables are called variables because they vary . The idea is that the contents, or values, of these variables will change as users interact with the programs you write.

